Javascript Password Meter
This code is for password meter as you see in gmail that measure the password srength...
Copy the Bellow code and run...
<html>
<head runat="server">
<title></title>
<script type="text/javascript" language="javascript">
function pass(ctrl)
{
if (document.getElementById("passdiv") == null)
{
var newPos = getPosition(ctrl);
var coods = newPos.split(" ");
var leftPos = parseInt(coods[0]);
var topPos = parseInt(coods[1]);
var passDiv = document.createElement("div");
passDiv.setAttribute("id", "passdiv");
passDiv.style.left = (leftPos + 160) + 'px';
passDiv.style.top = (topPos + 15) + 'px';
passDiv.style.width = '1px';
passDiv.style.height = '5px';
passDiv.style.position = 'absolute';
passDiv.style.background = 'Red';
document.body.appendChild(passDiv);
}
var divId = document.getElementById("passdiv");
var val = ctrl.value;
var divWidth = parseInt(divId.style.width);
if (val.length > 6)
{
divId.style.background = 'Yellow';
}
if (val.length > 10)
{
divId.style.background = 'Green';
}
if (parseInt(divId.style.width) < 130)
{
divId.style.width = (divWidth + parseInt(val.length)) + 'px';
setTimeout(function () { motion(parseInt(val.length) * 20); }, 100);
}
else
{
return;
}
}
function motion(val)
{
var divId = document.getElementById("passdiv");
var divWidth = parseInt(divId.style.width);
if (divWidth < parseInt(val))
{
setTimeout(function ()
{
divId.style.width = parseInt(divWidth + 3) + 'px';
motion(val);
}, 10);
}
else
{
return;
}
}
function getPosition(obj)
{
var topValue = 0;
var leftValue = 0;
while (obj)
{
leftValue += obj.offsetLeft;
topValue += obj.offsetTop;
obj = obj.offsetParent;
}
var finalValue = leftValue + " " + topValue;
return finalValue;
}
</script>
</head>
<body>
<form id="form1" runat="server">
<div>
<input type="text" id="passwd" onkeyup="pass(this);" />
</div>
<br />
</form>
</body>
</html>
Screenshot:-
Download the complete code form here
http://cid-fd367ee904a1f99a.office.live.com/self.aspx/Javascript/passwordmeter.rar
Copy the Bellow code and run...
<html>
<head runat="server">
<title></title>
<script type="text/javascript" language="javascript">
function pass(ctrl)
{
if (document.getElementById("passdiv") == null)
{
var newPos = getPosition(ctrl);
var coods = newPos.split(" ");
var leftPos = parseInt(coods[0]);
var topPos = parseInt(coods[1]);
var passDiv = document.createElement("div");
passDiv.setAttribute("id", "passdiv");
passDiv.style.left = (leftPos + 160) + 'px';
passDiv.style.top = (topPos + 15) + 'px';
passDiv.style.width = '1px';
passDiv.style.height = '5px';
passDiv.style.position = 'absolute';
passDiv.style.background = 'Red';
document.body.appendChild(passDiv);
}
var divId = document.getElementById("passdiv");
var val = ctrl.value;
var divWidth = parseInt(divId.style.width);
if (val.length > 6)
{
divId.style.background = 'Yellow';
}
if (val.length > 10)
{
divId.style.background = 'Green';
}
if (parseInt(divId.style.width) < 130)
{
divId.style.width = (divWidth + parseInt(val.length)) + 'px';
setTimeout(function () { motion(parseInt(val.length) * 20); }, 100);
}
else
{
return;
}
}
function motion(val)
{
var divId = document.getElementById("passdiv");
var divWidth = parseInt(divId.style.width);
if (divWidth < parseInt(val))
{
setTimeout(function ()
{
divId.style.width = parseInt(divWidth + 3) + 'px';
motion(val);
}, 10);
}
else
{
return;
}
}
function getPosition(obj)
{
var topValue = 0;
var leftValue = 0;
while (obj)
{
leftValue += obj.offsetLeft;
topValue += obj.offsetTop;
obj = obj.offsetParent;
}
var finalValue = leftValue + " " + topValue;
return finalValue;
}
</script>
</head>
<body>
<form id="form1" runat="server">
<div>
<input type="text" id="passwd" onkeyup="pass(this);" />
</div>
<br />
</form>
</body>
</html>
Screenshot:-
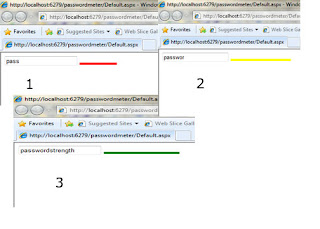
Download the complete code form here
http://cid-fd367ee904a1f99a.office.live.com/self.aspx/Javascript/passwordmeter.rar
Comments
Post a Comment